Add a User with View Access to a Google Drive File
Posted By: Harish
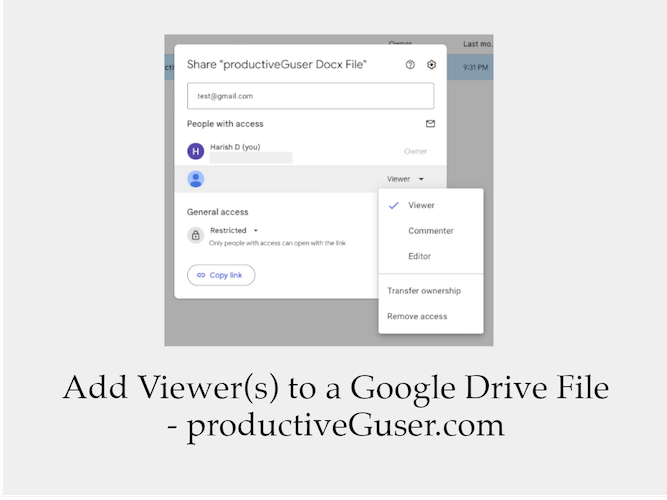
We have seen ways to get a list of users with view access for a file or a folder.
Now, in this post, we will see different ways to add user email with only view access to a file.
Add an email to the file using google drive website
Open our Google Drive and visit the parent folder of the file.
Select the file to get extra options at the top of the folder.
Click on the person icon with plus symbol
(on hover you will get Share
overlay text).
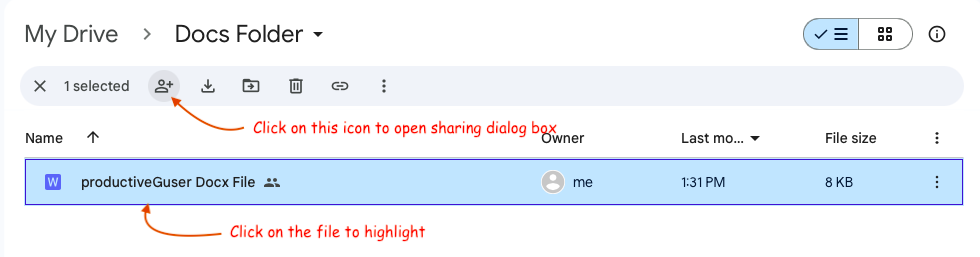
A pop up dialog box will open with info about the shared users and file permissions.
Enter the email in the input box to give user file permissions. That's it. Your file will be shared with the user.
Choose the Viewer
option to give view access.
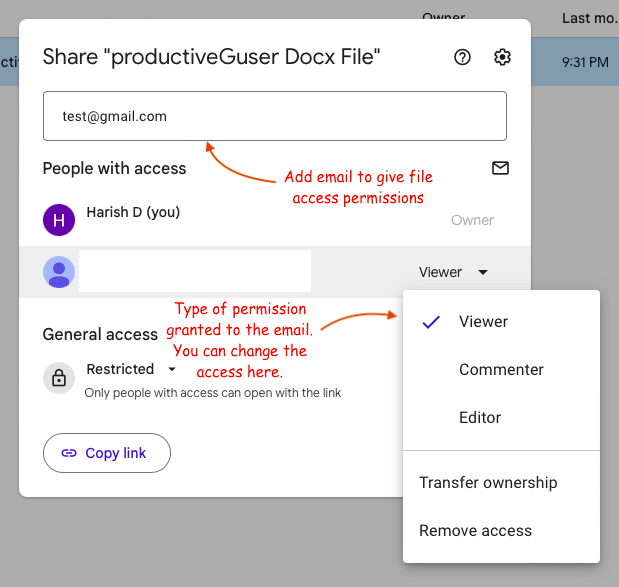
You can also visit the shared dialog box by clicking on three dots of the file and selecting Share
option.
Add an email with viewing permission using Google Apps Script when file name is known
Open Google Apps Script editor and create a new project.
Replace the default function (which already exists) with the below function and run it. Visit creating and executing a Google Apps Script post for more info.
function AddSingleFileViewerWhenFileNameIsKnown() {
try {
const userEmail = '[email protected]'; //<-- replace the user email address here
const fileName = 'productiveGuser Docx File' //<-- replace your file name here
const files = DriveApp.getFilesByName(fileName);
while (files.hasNext()) {
const file = files.next();
const getAccess = file.getAccess(userEmail);
const accessType = getAccess.toString();
if (accessType == DriveApp.Permission.COMMENT) {
file.removeCommenter(userEmail)
} else if (accessType == DriveApp.Permission.EDIT) {
file.removeViewer(userEmail)
};
const updatedFile = file.addViewer(userEmail);
const updatedAccess = updatedFile.getAccess(userEmail);
const updatedAccessType = updatedAccess.toString();
const fileUrl = updatedFile.getUrl()
console.log(`updated Access of ${userEmail}: `, updatedAccessType)
console.log('File Link: ', fileUrl)
}
} catch (err) {
Logger.log(err)
}
}
Run above script to give view access to a single user.
This script gets a list of files with the given file name and checks if the given email has already any other access permissions.
Like if the email already has commenter or editor permissions, we have to remove those permissions to give view permission.
We can change the user permissions using Google Drive API without deleting them, but that is beyond the scope of this article. We will cover that in future articles.
After removing access, we will add the user again with viewer access using addViewer method.
Add an email with viewing permission using Google Apps Script when file ID is known
If you don't know the file name but have the file link, then extract the file ID from the link, replace the file ID in below script and run it.
function AddSingleFileViewerWhenFileLinkIsKnown() {
try {
const userEmail = '[email protected]'; //<-- replace the user email address here
const fileId = '1-QdZbhH622i58fEoeMRQ_6TnbeH12345' //<-- replace file ID here
const file = DriveApp.getFileById(fileId);
const getAccess = file.getAccess(userEmail);
const accessType = getAccess.toString();
if (accessType == DriveApp.Permission.COMMENT) {
file.removeCommenter(userEmail)
} else if (accessType == DriveApp.Permission.EDIT) {
file.removeViewer(userEmail)
};
const updatedFile = file.addViewer(userEmail);
const updatedAccess = updatedFile.getAccess(userEmail);
const updatedAccessType = updatedAccess.toString();
const fileUrl = updatedFile.getUrl()
console.log(`updated Access of ${userEmail}: `, updatedAccessType)
console.log('File Link: ', fileUrl)
} catch (err) {
Logger.log(err)
}
}
This script is similar to above one, but in this we are getting a single file using its ID and adding the user. Best for adding a viewer to a specific file.
Add multiple emails at once with view permission using Google Apps Script when file name is known and uncertain about any permissions of those emails list
Run this script when you have multiple emails and don't know whether few or all users already have access to the file.
function AddMultipleViewersToFileUsingEmailListWhenFileNameIsKnown() {
try {
const emailList = [
'[email protected]',
'[email protected]',
]; //<-- replace with new emails.Add each email in new line and don't forget comma
const fileName = 'productiveGuser Docx File' //<-- replace file name here
const files = DriveApp.getFilesByName(fileName);
while (files.hasNext()) {
const file = files.next();
const fileUrl = file.getUrl()
emailList.forEach(email => {
const getAccess = file.getAccess(email);
const accessType = getAccess.toString();
if (accessType == DriveApp.Permission.COMMENT) {
file.removeEditor(email)
} else if (accessType == DriveApp.Permission.EDIT) {
file.removeViewer(email)
};
const updatedFile = file.addViewer(email);
const updatedAccess = updatedFile.getAccess(email);
const updatedAccessType = updatedAccess.toString();
console.log(`updated Access of ${email}: `, updatedAccessType);
});
console.log('file link: ', fileUrl)
}
} catch (err) {
Logger.log(err)
}
}
Here we are looping the email list to check for previous permissions and remove them if any.
After that, an individual email is added using the addViewer
method.
Add multiple emails at once with view permission using Google Apps Script when file ID is known and uncertain about any permissions of those emails list
If you have only file ID, then run below script by replacing the emails and file ID.
function AddMultipleViewersToFileUsingEmailListWhenFileIdIsKnown() {
try {
const emailList = [
'[email protected]',
'[email protected]',
]; //<-- replace with new emails.Add each email in new line and don't forget comma
const fileId = '1-QdZbhH622i58fEoeMRQ_6TnbeH12345' //<-- replace file ID here
const file = DriveApp.getFileById(fileId);
emailList.forEach(email => {
const getAccess = file.getAccess(email);
const accessType = getAccess.toString();
if (accessType == DriveApp.Permission.COMMENT) {
file.removeEditor(email)
} else if (accessType == DriveApp.Permission.EDIT) {
file.removeViewer(email)
};
const updatedFile = file.addViewer(email);
const updatedAccess = updatedFile.getAccess(email);
const updatedAccessType = updatedAccess.toString();
console.log(`updated Access of ${email}: `, updatedAccessType);
});
} catch (err) {
Logger.log(err)
}
}
Add multiple emails at once with view permission using Google Apps Script when file name is known and emails are new to file permissions
If the emails are new to file permissions then you can use below script to add viewers without checking for any previous permissions.
function AddMultipleFileViewersUsingNewEmailsWhenFileNameIsKnown() {
try {
const emailList = [
'[email protected]',
'[email protected]',
]; //<-- replace with new emails.Add each email in new line and don't forget comma
const fileName = 'productiveGuser Docx File' //<-- replace file name here
const files = DriveApp.getFilesByName(fileName);
while (files.hasNext()) {
const file = files.next();
const updatedFile = file.addViewers(emailList);
const fileUrl = updatedFile.getUrl()
console.log('File Link: ', fileUrl);
}
} catch (err) {
Logger.log(err)
}
}
In this script, we are using the new Google Drive method, addViewers to add multiple emails at once.
Add multiple emails at once with view permission using Google Apps Script when file ID is known and emails are new to file permissions
Run below script when file ID is known.
function AddMultipleFileViewersUsingNewEmailsWhenFileIdIsKnown() {
try {
const emailList = [
'[email protected]',
'[email protected]',
]; //<-- replace with new emails.Add each email in new line and don't forget comma
const fileId = '1-QdZbhH622i58fEoeMRQ_6TnbeH12345' //<-- replace file ID here
const file = DriveApp.getFileById(fileId);
const updatedFile = file.addViewers(emailList);
const fileUrl = updatedFile.getUrl()
console.log('File Link: ', fileUrl);
} catch (err) {
Logger.log(err)
}
}
After successful execution of these scripts, you can check the file sharing permission by visiting the google drive.
These scripts can be used by the owner of the file or other users with editor access.
So, above are a few ways to give viewing permissions to an email or multiple emails at once.