Add a User with View Access to a Google Drive Folder
Posted By: Harish
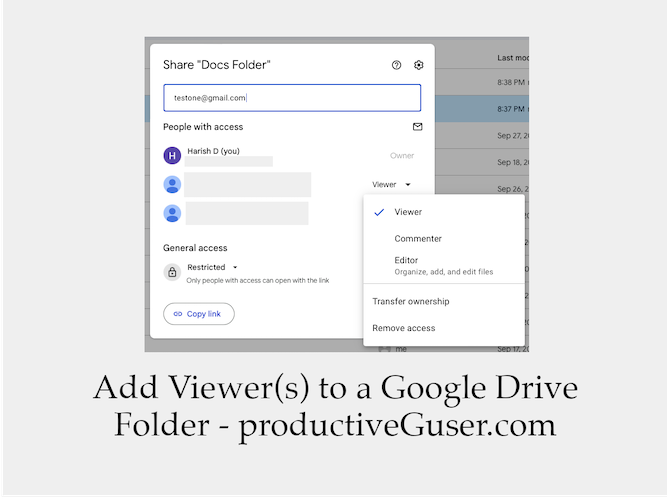
We have seen a few ways to give a user view permissions to a file.
Now, in this post, we will look into ways to add user(s) with view access to a folder.
The process of giving view access is similar to that of a file. But when we add a user to a folder with view access, that user can view files and inner folders of that folder without giving any individual access to files or inner folders.
Add an email to the file using google drive website
Open our Google Drive and click on My Drive option (Situated at left side of the screen when opened in a computer browser or directly click on above My Drive link to open).
Search for the folder and select it to highlight the extra options at the top of the page.
Click on the person icon with plus symbol
(on hover you will get Share
overlay text).
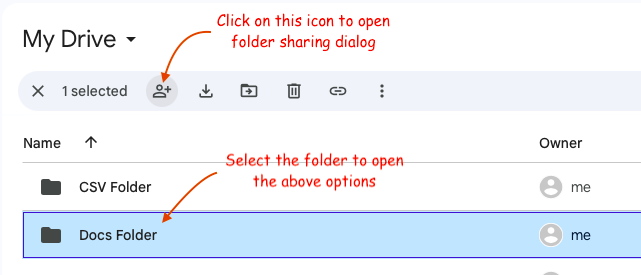
A pop up dialog box will open with info about the added users and their permissions.
Enter the email in the input box to give user folder access permissions. That's it. Your folder will be shared with the user.
Choose the Viewer
option to give view access to the email.
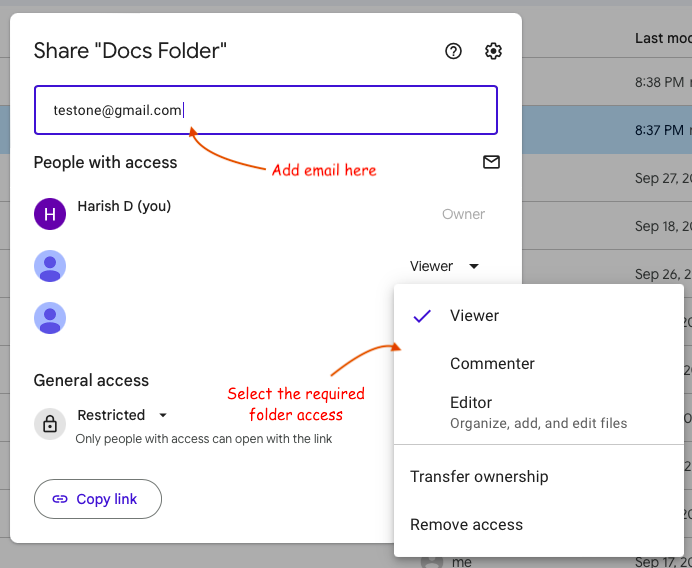
You can also visit the shared dialog box by clicking on three dots of the folder and selecting Share
option.
Add an email with viewing permission using Google Apps Script when folder name is known
Open Google Apps Script editor and create a new project.
Replace the default function (which already exists) with the below function and run it. Visit creating and executing a Google Apps Script post for more info.
function AddSingleFolderViewerWhenFolderNameIsKnown() {
try {
const userEmail = '[email protected]'; //<-- replace the user email address here
const folderName = 'Docs Folder' //<-- replace your folder name here
const folders = DriveApp.getFoldersByName(folderName);
while (folders.hasNext()) {
const folder = folders.next();
const getAccess = folder.getAccess(userEmail);
const accessType = getAccess.toString();
if (accessType == DriveApp.Permission.COMMENT) {
folder.removeCommenter(userEmail)
} else if (accessType == DriveApp.Permission.EDIT) {
folder.removeViewer(userEmail)
};
const updatedFolder = folder.addViewer(userEmail);
const updatedAccess = updatedFolder.getAccess(userEmail);
const updatedAccessType = updatedAccess.toString();
const folderLink = updatedFolder.getUrl()
console.log(`updated Access of ${userEmail}: `, updatedAccessType)
console.log('Folder Link: ', folderLink)
}
} catch (err) {
Logger.log(err)
}
}
Run above script to give view access to a single user.
This script gets a list of all folders with the given folder name and checks if the given email has already any other access permissions.
Like, if the email already has commenter or editor permissions, we have to remove those permissions to give view permission.
We can change the user permissions using Google Drive API without deleting them, but that is beyond the scope of this article. We will cover that in future articles.
After removing access, we will add the user again with viewer access using addViewer method.
Add an email with viewing permission using Google Apps Script when folder ID is known
If you don't know the folder name but have the folder link, then extract the folder ID from the link, replace the folder ID in below script and run it.
function AddSingleFolderViewerWhenFolderLinkIsKnown() {
try {
const userEmail = '[email protected]'; //<-- replace the user email address here
const folderId = '1QuXvSRAJX9hHyijdgcEGWgEl7Bm12345' //<-- replace folder ID here
const folder = DriveApp.getFolderById(folderId);
const getAccess = folder.getAccess(userEmail);
const accessType = getAccess.toString();
if (accessType == DriveApp.Permission.COMMENT) {
folder.removeCommenter(userEmail)
} else if (accessType == DriveApp.Permission.EDIT) {
folder.removeViewer(userEmail)
};
const updatedFolder = folder.addViewer(userEmail);
const updatedAccess = updatedFolder.getAccess(userEmail);
const updatedAccessType = updatedAccess.toString();
const folderLink = updatedFolder.getUrl()
console.log(`updated Access of ${userEmail}: `, updatedAccessType)
console.log('Folder Link: ', folderLink)
} catch (err) {
Logger.log(err)
}
}
This script is similar to above one, but in this we are getting a single folder using its ID and are adding the user. Best for adding a viewer to a specific folder.
Add multiple emails at once with view permission using Google Apps Script when folder name is known and uncertain about any permissions of those emails list
Run this script when you have multiple emails and don't know whether few or all users already have access to the folder.
function AddMultipleViewersToFolderUsingEmailListWhenFolderNameIsKnown() {
try {
const emailList = [
'[email protected]',
'[email protected]',
]; //<-- replace with new emails.Add each email in new line and don't forget comma
const folderName = 'Docs Folder' //<-- replace your folder name here
const folders = DriveApp.getFoldersByName(folderName);
while (folders.hasNext()) {
const folder = folders.next();
const folderUrl = folder.getUrl()
emailList.forEach(email => {
const getAccess = folder.getAccess(email);
const accessType = getAccess.toString();
if (accessType == DriveApp.Permission.COMMENT) {
folder.removeEditor(email)
} else if (accessType == DriveApp.Permission.EDIT) {
folder.removeViewer(email)
};
const updatedFolder = folder.addViewer(email);
const updatedAccess = updatedFolder.getAccess(email);
const updatedAccessType = updatedAccess.toString();
console.log(`updated Access of ${email}: `, updatedAccessType);
});
console.log('Folder Link: ', folderUrl)
}
} catch (err) {
Logger.log(err)
}
}
In this, we are looping the email list to check for previous permissions and remove them if any.
After that, an individual email is added using the addViewer
method.
Add multiple emails at once with view permission using Google Apps Script when folder ID is known and uncertain about any permissions of those emails list
If you have only folder ID, then run below script by replacing the emails and folder ID.
function AddMultipleViewersToFolderUsingEmailListWhenFolderIdIsKnown() {
try {
const emailList = [
‘testone@gmail.com',
‘[email protected]',
]; //<-- replace with new emails.Add each email in new line and don't forget comma
const folderId = '1QuXvSRAJX9hHyijdgcEGWgEl7Bm12345' //<-- replace folder ID here
const folder = DriveApp.getFolderById(folderId);
const folderLink = folder.getUrl()
emailList.forEach(email => {
const getAccess = folder.getAccess(email);
const accessType = getAccess.toString();
if (accessType == DriveApp.Permission.COMMENT) {
folder.removeEditor(email)
} else if (accessType == DriveApp.Permission.EDIT) {
folder.removeViewer(email)
};
const updatedFolder = folder.addViewer(email);
const updatedAccess = updatedFolder.getAccess(email);
const updatedAccessType = updatedAccess.toString();
console.log(`updated Access of ${email}: `, updatedAccessType);
});
console.log('Folder Link: ', folderLink)
} catch (err) {
Logger.log(err)
}
}
Add multiple emails at once with view permission using Google Apps Script when folder name is known and emails are new to folder permissions
If the emails are new to folder permissions then you can use below script to add viewers without checking for any previous permissions.
function AddMultipleFolderViewersUsingNewEmailsWhenFolderNameIsKnown() {
try {
const emailList = [
‘testone@gmail.com',
‘[email protected]',
]; //<-- replace with new emails.Add each email in new line and don't forget comma
const folderName = 'Docs Folder' //<-- replace your folder name here
const folders = DriveApp.getFoldersByName(folderName);
while (folders.hasNext()) {
const folder = folders.next();
const updatedFolder = folder.addViewers(emailList);
const folderLink = updatedFolder.getUrl()
console.log('Folder Link: ', folderLink);
}
} catch (err) {
Logger.log(err)
}
}
In this script, we are using a new Google Drive method, addViewers to add multiple emails at once.
Add multiple emails at once with view permission using Google Apps Script when folder ID is known and emails are new to folder permissions
Run below script when folder ID is known.
function AddMultipleFolderViewersUsingNewEmailsWhenFolderIdIsKnown() {
try {
const emailList = [
‘testone@gmail.com',
‘[email protected]',
]; //<-- replace with new emails.Add each email in new line and don't forget comma
const folderId = ‘1QuXvSRAJX9hHyijdgcEGWgEl7Bm12345’ //<-- replace folder ID here
const folder = DriveApp.getFolderById(folderId);
const updatedFolder = folder.addViewers(emailList);
const folderUrl = updatedFolder.getUrl()
console.log('Folder Link: ', folderUrl);
} catch (err) {
Logger.log(err)
}
}
After successful execution of these scripts, you can check the folder sharing permission by visiting the google drive.
These scripts can be used by the owner of the folder or other users with editor access.
So, above are a few ways to give viewing permissions to an email or multiple emails at once.