Get a List of All Google Drive Files
Posted By: Harish
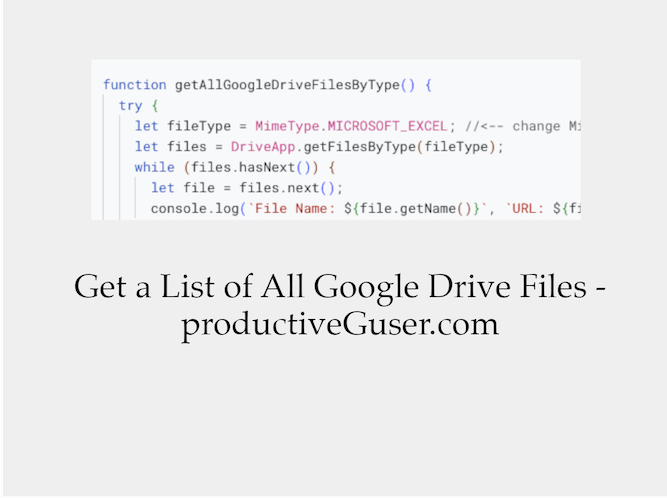
Sometimes, we may want a list of files in our drive or even specific types like excel or google sheet documents stored in our drive.
In those scenarios, we can use below listed scripts to get the list of all google drive files stored in different folders.
Get a list of file names stored in google drive
Open Google Apps Script editor and create a new project.
Replace the default function (which already exists) with the below function and run it. Visit creating and executing a Google Apps Script post for more info.
function getAllGoogleDriveFiles() {
try {
const files = DriveApp.getFiles();
while (files.hasNext()) {
const file = files.next();
console.log(`File Name: ${file.getName()}`, `URL: ${file.getUrl()}`);
}
} catch (err) {
Logger.log(err)
}
}
Run the above function to get a list of all documents, sheets, files etc., in the execution log window.
This list includes all files of google drive and its folders.
Get list of all files with same file name
If you have multiple files with the same file name, run the below function to get the list of all file links with the same name.
function getAllGoogleDriveFilesByName() {
try {
let fileName = 'Untitled Project' //<-- change file name here
let files = DriveApp.getFilesByName(fileName);
while (files.hasNext()) {
let file = files.next();
console.log(`File URL: ${file.getUrl()}`);
}
} catch (err) {
Logger.log(err)
}
}
Get a list of all files in a google drive folder
Above, we have a method to get all file names and their links stored on our google drive.
Now, to get a list of files stored in a specific folder, execute below command.
function getAllFilesOfAGoogleDriveFolder() {
try {
let folderName = 'Excel Folder';
let files = DriveApp.getFoldersByName(folderName);
while (files.hasNext()) {
let fileFolder = files.next();
let folderFiles = fileFolder.getFiles();
while (folderFiles.hasNext()) {
let file = folderFiles.next();
console.log(`File Name: ${file.getName()}`, `URL: ${file.getUrl()}`);
}
}
} catch (err) {
Logger.log(err)
}
}
Get a list of same type files stored in google drive
Below function prints the list of same MimeType files like a list of all excel sheets, google docs etc.
function getAllGoogleDriveFilesByType() {
try {
let fileType = MimeType.MICROSOFT_EXCEL; //<-- Change MimeType here
let files = DriveApp.getFilesByType(fileType);
while (files.hasNext()) {
let file = files.next();
console.log(`File Name: ${file.getName()}`, `URL: ${file.getUrl()}`);
}
} catch (err) {
Logger.log(err)
}
}
You can get other MimeTypes by visiting Google Apps Script MimeTypes.
Get a list of same MimeType files stored in a google drive folder
Below function prints the list of all files in a folder with the same MimeType.
function getAllSameTypeFilesOfAFolder() {
try {
let folderName = 'Excel Folder';
let fileType = MimeType.MICROSOFT_EXCEL;
let folders = DriveApp.getFoldersByName(folderName);
while (folders.hasNext()) {
let fileFolder = folders.next();
let folderFiles = fileFolder.getFilesByType(fileType);
while (folderFiles.hasNext()) {
let file = folderFiles.next();
console.log(`File Name: ${file.getName()}`, `URL: ${file.getUrl()}`);
}
}
} catch (err) {
Logger.log(err)
}
}
So, above are a few scripts to get a list of files stored in google drive and its folder.