Get the Users with View Access of a Google Drive File
Posted By: Harish
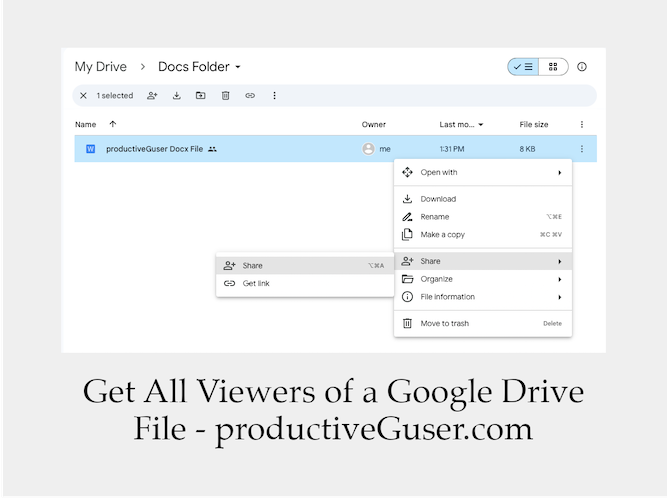
A user who has a View
access rights for a google drive file, can only view it and misses other privileges like commenting, editing or sharing. You can find full list of what viewers can do HERE.
If the file has general sharing access to any one with the link, then everyone will become a viewer, even without adding their email to access the file.
Lets see different ways to get the users with view permissions of a private file.
Get all viewers by opening google drive app or website
This is the basic and general way to check all viewers of a private file.
Open our Google Drive and visit the parent folder of the file.
Select the file to get extra options at the top of the folder.
Click on the person icon with plus symbol
(on hover you will get Share
overlay text).
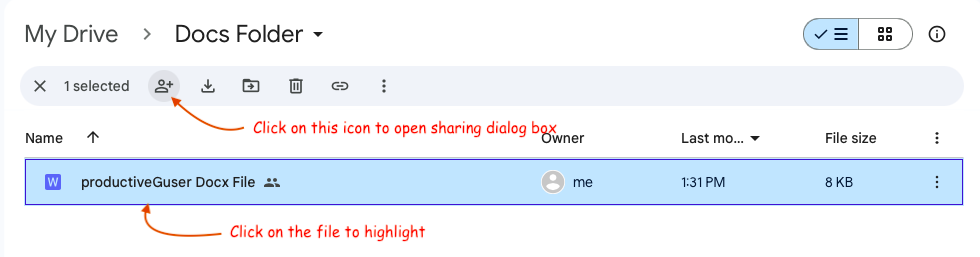
A pop up dialog box will open with info about the added users and file's sharing permissions.
The emails which have the Viewer
as selected dropdown option, are the viewers of the file.
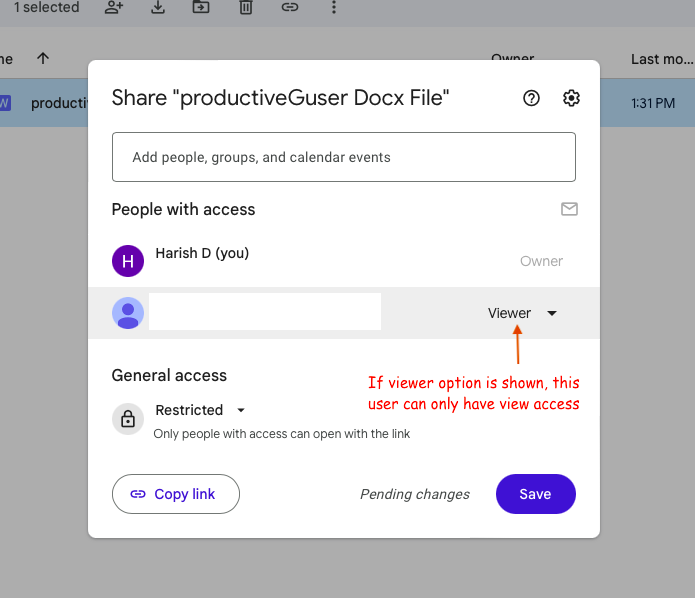
You can also visit the shared dialog box by clicking on three dots of the file and selecting Share
option.
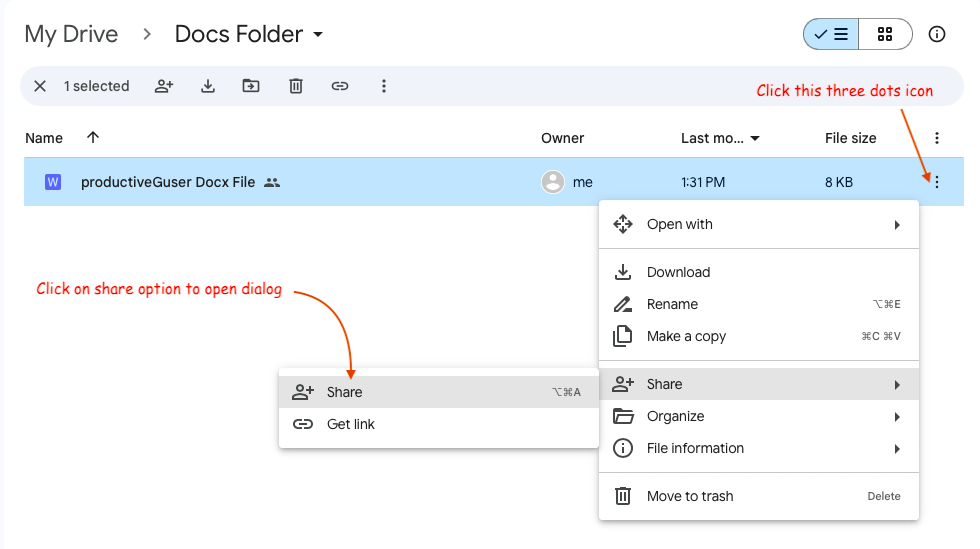
This process is the same for a folder too.
But when we give access to a folder, the viewer will automatically have access to the files and internal folders of that folder.
Get all google drive file viewers using Google Apps Script when file name is known
Open Google Apps Script editor and create a new project.
Replace the default function (which already exists) with the below function and run it. Visit creating and executing a Google Apps Script post for more info.
function getAllViewersOfAFileWhenFileNameIsKnown() {
try {
const fileName = 'productiveGuser Docx File'; //replace your file name here
const files = DriveApp.getFilesByName(fileName);
while (files.hasNext()) {
const file = files.next();
const fileUrl = file.getUrl();
const viewersList = file.getViewers();
if (viewersList.length === 0) {
console.log('No Viewers for this file');
return;
};
for (let i = 0; i < viewersList.length; i++) {
const viewer = viewersList[i];
const viewerName = viewer.getName();
const viewerEmail = viewer.getEmail();
console.log(`Name: ${viewerName}, Email: ${viewerEmail}`);
};
console.log('File Link: ', fileUrl)
}
} catch (err) {
Logger.log(err)
}
}
Above function checks for the file in the drive and gets the viewers list.
Keep note that this script gets the viewers of all files which are having the same name.
Get all google drive file viewers using Google Apps Script when file link or file ID is known
If you don't have the file name but have the link to the file, then you can get the file using file id. This is the best way to get viewers of a specific file. For more info on getting google drive file ID, check get google drive file id post.
function getAllViewersOfAFileWhenFileLinkIsKnown() {
try {
const fileId = '1-QdZbhH622i58fEoeMRQ_6TnbeHl7777'; //replace your file ID here
const file = DriveApp.getFileById(fileId);
const viewersList = file.getViewers();
if (viewersList.length === 0) {
console.log('No Viewers for this file');
return;
};
for (let i = 0; i < viewersList.length; i++) {
const viewer = viewersList[i];
const viewerName = viewer.getName();
const viewerEmail = viewer.getEmail();
console.log(`Name: ${viewerName}, Email: ${viewerEmail}`);
};
} catch (err) {
Logger.log(err)
}
}
Run above function when you have a file link or file ID and want to know users with viewing permissions for a specific file.
These scripts should run by the owner or any user with editor permissions.
So, in this way we can get a list of all viewers of a private google drive file.