Get the Users with View Access of a Google Drive Folder
Posted By: Harish
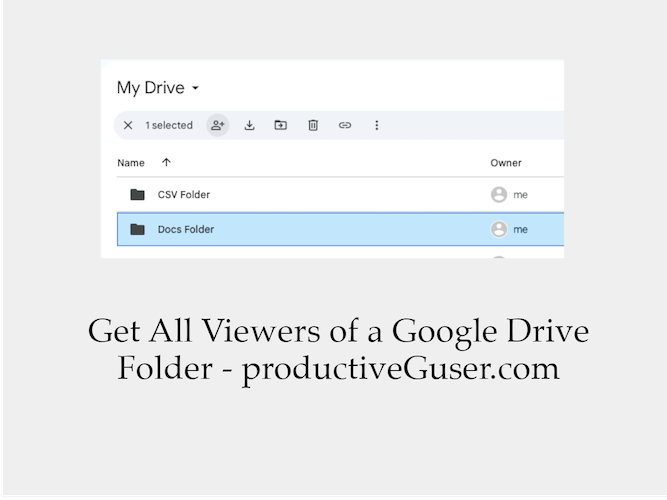
We have seen a way to get a list of users with view permissions for a google drive file.
In this post, we will check for viewers of a folder. A google drive folder viewer will have access to view the folder and its content.
The process is the same for file and folder but the only difference is that the user can access every file and internal folders of that folder.
Lets see different ways to check the viewers of a folder.
Get all viewers by opening google drive app or website
This is the basic and general way to check all viewers of a private file.
Open our Google Drive and click on My Drive option (Situated at left side of the screen when opened in a computer browser or directly click on above My Drive link to open).
Search for the folder and select it to highlight the extra options at the top of the page.
Click on the person icon with plus symbol
(on hover you will get Share
overlay text).
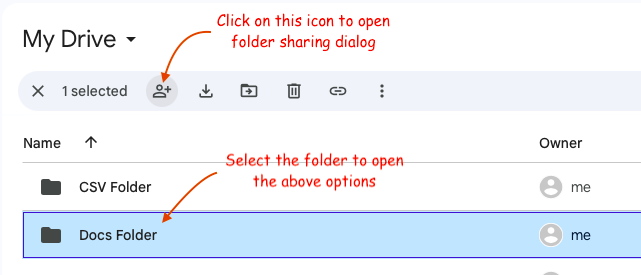
A pop up dialog box will open with info about the added users and their permissions.
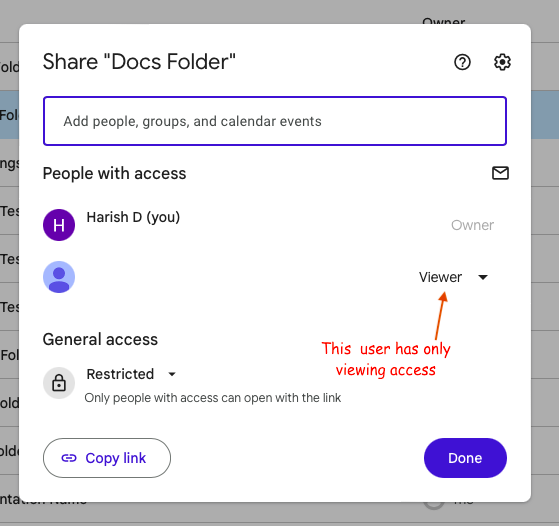
The emails which have the Viewer
option as selected are the viewers of the file, meaning they can only view the file.
You can also visit the sharing dialog box by clicking on three dots of the folder and selecting Share
option.
Get all google drive folder viewers using Google Apps Script when folder name is known
Open Google Apps Script editor and create a new project.
Replace the default function (which already exists) with the below function and run it. Visit creating and executing a Google Apps Script post for more info.
function getAllViewersOfAFolderWhenFolderNameIsKnown() {
try {
const folderName = 'Docs Folder'; //replace your folder name here
const folders = DriveApp.getFoldersByName(folderName);
while (folders.hasNext()) {
const folder = folders.next();
const folderUrl = folder.getUrl();
const viewersList = folder.getViewers();
if (viewersList.length === 0) {
console.log('No Viewers for this folder');
return;
};
for (let i = 0; i < viewersList.length; i++) {
const viewer = viewersList[i];
const viewerName = viewer.getName();
const viewerEmail = viewer.getEmail();
console.log(`Name: ${viewerName}, Email: ${viewerEmail}`);
};
console.log('Folder Link: ', folderUrl)
}
} catch (err) {
Logger.log(err)
}
}
Above function checks for the folder in the drive and gets the viewers list.
Keep note that this script gets the viewers of all folders which are having the same name.
Get all folder viewers using Google Apps Script when folder link or folder ID is known
If you don't have the folder name but have the folder link, then you can get the folder using folder id. This is the best way to get viewers of a specific folder. For more info on getting google drive folder ID, check get google drive folder id post.
function getAllViewersOfAFolderWhenFolderLinkIsKnown() {
try {
const folderId = '1olZJLpCC_U2aFv-Y0PLkkskJdVuVDqEOK'; //replace your folder ID here
const folder = DriveApp.getFolderById(folderId);
const viewersList = folder.getViewers();
if (viewersList.length === 0) {
console.log('No Viewers for this folder');
return;
};
for (let i = 0; i < viewersList.length; i++) {
const viewer = viewersList[i];
const viewerName = viewer.getName();
const viewerEmail = viewer.getEmail();
console.log(`Name: ${viewerName}, Email: ${viewerEmail}`);
};
} catch (err) {
Logger.log(err)
}
}
Run above function when you have a folder link or folder ID and want to know users with viewing permissions for a specific folder.
These scripts should run by the owner or any user with editor permissions.
So, in this way we can get a list of all viewers of a private google drive folder.