Get the List of Google Drive Folders
Posted By: Harish
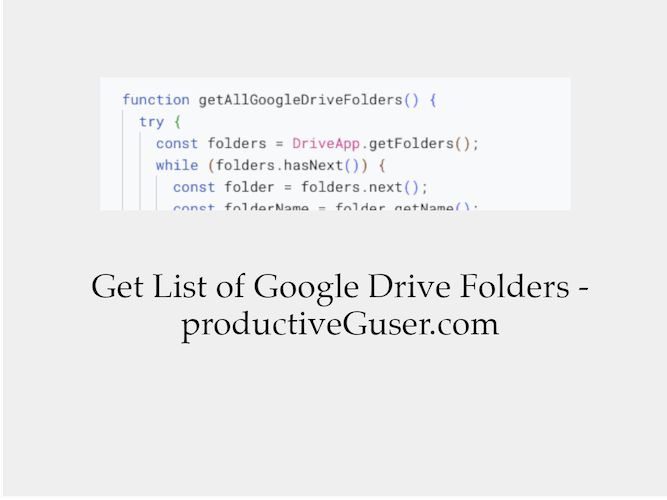
We know to get a google drive folder ID from its link or using a script. But what if we want a link from the folder name or a list of all folders and their links?
In this post, we will discuss ways to get a google drive folder or folders using scripts.
Get a List of All Google Drive Folders
Open Google Apps Script editor and create a new project.
Replace the default function (which already exists) with the below function and run it. Visit creating and executing a Google Apps Script post for more info.
function getAllGoogleDriveFolders() {
try {
const folders = DriveApp.getFolders();
while (folders.hasNext()) {
const folder = folders.next();
const folderName = folder.getName();
const folderLink = folder.getUrl()
console.log(`Name: ${folderName} , Link: ${folderLink}`);
}
} catch (err) {
Logger.log(err)
}
}
Run the above function to get all folder names and their links in the execution log window.
Get Google Drive Folder Link using its Name
If you know the name of the folder, we can get the link by running the below function.
function getAllGoogleDriveFoldersByName() {
try {
const folderName = "Drive Test" //<-- replace your folder name here
const folders = DriveApp.getFoldersByName(folderName);
while (folders.hasNext()) {
const folder = folders.next();
const folderName = folder.getName();
const folderLink = folder.getUrl()
console.log(`Name: ${folderName} , Link: ${folderLink}`);
}
} catch (err) {
Logger.log(err)
}
}
By executing the above function, you will get the folder or folders having the same name with their links in the execution log window.
Get Google Drive Folder Name and its Link using its Folder ID
If you have a link to the folder, we can get the name of the folder by running the below method.
For this, we need to extract the folder ID from the link. You can check getting a google drive folder ID post for more info.
function getGoogleDriveFolderNameById() {
try {
const folderId = "1QuXvSRAJX9hHyijdgcEGWgEl7Bmlcuiz" //<-- replace your folder ID here
const folder = DriveApp.getFolderById(folderId);
const folderName = folder.getName();
const folderLink = folder.getUrl()
console.log(`Name: ${folderName} , Link: ${folderLink}`);
} catch (err) {
Logger.log(err)
}
}
So, these are a few ways to get the folders of your google drive.